The Python Assert Statement
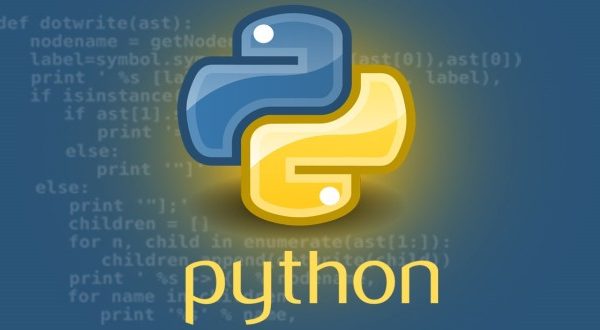
In this article, we’ll examine how to use the assert
statement in Python.
In Python, the assert
statement is used to validate whether or not a condition is true, using the syntax:
assert
If the condition evaluates to True
, the program continues executing as if nothing out of the ordinary happened. However, if the condition evaluates to False
, the program terminates with an AssertionError
.
>>> assert True
Nothing happens when the code above is executed, since the condition evaluates to True
. Alternatively, the condition in the example below evaluates to False
:
>>> assert False
Traceback (most recent call last):
File "", line 1, in
AssertionError
For added clarity, we can add a custom error message to the assertion output as follows:
>>> assert False, "This is a custom assertion message!"
Traceback (most recent call last):
File "", line 1, in
AssertionError: This is a custom assertion message!
The assert statement is useful when we want to check that a variable in our code assumes the correct value and terminate the program if it doesn’t. This helps prevent silent failure modes, which can occur if the program continues executing