Python based SDK for multi human pose estimation through RGB webcam
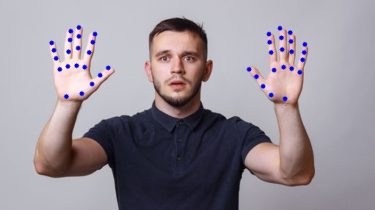
PoseCamera
PoseCamera is python based SDK for multi human pose estimation through RGB webcam.
Install
install posecamera package through pip
pip install posecamera
If you are having issues with the installation on Windows OS then check this page
Usage
See Google colab notebook https://colab.research.google.com/drive/18uoYeKmliOFV8dTdOrXocClCA7nTwRcX?usp=sharing
draw pose keypoints on image
import posecamera
import cv2
det = posecamera.pose_tracker.PoseTracker()
image = cv2.imread("example.jpg")
pose = det(image)
for name, (y, x, score) in pose.keypoints.items():
cv2.circle(image, (int(x), int(y)), 4, (255, 0, 0), -1)
cv2.imshow("PoseCamera", image)
cv2.waitKey(0)
output of the above example
or get keypoints array
for pose in poses:
keypoints = pose.keypoints
Handtracker
import posecamera
import cv2
det = posecamera.hand_tracker.HandTracker()
image = cv2.imread("tmp/hands.jpg")
keypoints, bbox = det(image)
for hand_keypoints in keypoints: