‘is’ vs ‘==’ in Python – Object Comparison
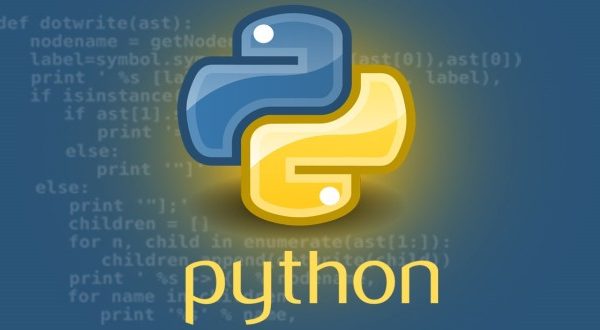
‘is’ vs ‘==’ in Python
Python has two very similar operators for checking whether two objects are equal. These two operators are is
and ==
.
They are usually confused with one another because with simple data types, like int
s and string
s (which many people start learning Python with) they seem to do the same thing:
x = 5
s = "example"
print("x == 5: " + str(x == 5))
print("x is 5: " + str(x is 5))
print("s == 'example': " + str(s == "example"))
print("s is 'example': " + str(s is "example"))
Running this code will result in:
x == 5: True
x is 5: True
s == 'example': True
s is 'example': True
This shows that ==
and is
return the same value (True
) in these cases. However, if you tried to do this with a more complicated structure:
some_list = [1]
print("some_list == [1]: " + str(some_list == [1]))
print("some_list is [1]: " + str(some_list is [1]))
This would result in:
some_list == [1]: True
some_list is [1]: False
Here it becomes obvious that these operators aren’t the same.
The difference comes from the fact