Convert Strings to Numbers and Numbers to Strings in Python
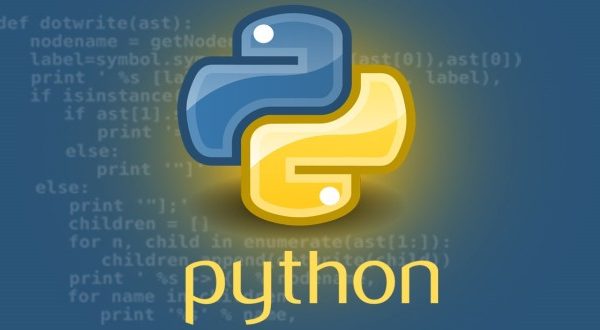
Introduction
Python allows you to convert strings, integers, and floats interchangeably in a few different ways. The simplest way to do this is using the basic str()
, int()
, and float()
functions. On top of this, there are a couple of other ways as well.
Before we get in to converting strings to numbers, and converting numbers to strings, let’s first see a bit about how strings and numbers are represented in Python.
Note: For simplicity of running and showing these examples we’ll be using the Python interpreter.
Strings
String literals in Python are declared by surrounding a character with double (“) or single quotation (‘) marks. Strings in Python are really just arrays with a Unicode for each character as an element in the array, allowing you to use indices to access a single character from the string.
For example, we can access individual characters of these strings by specifying an index:
>>> stringFirst = "Hello World!"
>>> stringSecond = 'Again!'
>>> stringFirst[3]
'l'
>>> stringSecond[3]
'i'
Numerics
A numeric in Python can be an integer
, a float
, or a complex
.
Integers can be a positive or negative whole number. Since Python 3, integers