Python List Sorting with sorted() and sort()
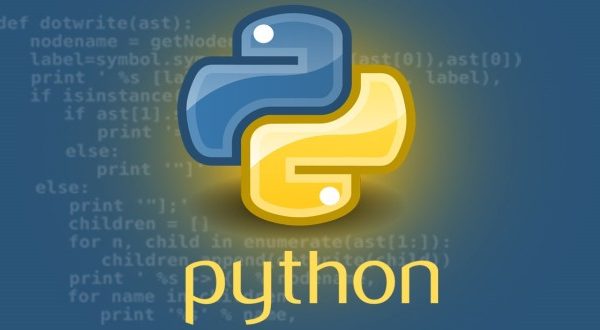
In this article, we’ll examine multiple ways to sort lists in Python.
Python ships with two built-in methods for sorting lists and other iterable objects. The method chosen for a particular use-case often depends on whether we want to sort a list in-place or return a new version of the sorted list.
Assuming we want to sort a list in place, we can use the list.sort()
method as follows:
>>> pets = ['Turtle', 'Cat', 'Fish', 'Dingo']
>>> pets.sort()
>>> pets
['Cat', 'Dingo', 'Fish', 'Turtle']
By default, the list is sorted in ascending order. Notice how the original pets list is changed after the sort method is called on it. If we don’t want this to happen, we can use the built-in sorted()
function to return a new sorted list while leaving the original list unchanged:
>>> pets = ['Turtle', 'Cat', 'Fish', 'Dingo']
>>> new_pets = sorted(pets)
>>> new_pets
['Cat', 'Dingo', 'Fish', 'Turtle']
>>> pets
['Turtle', 'Cat', 'Fish', 'Dingo']
The reverse argument can be used to sort lists in descending order:
>>> pets = ['Turtle', 'Cat', 'Fish', 'Dingo']
>>> new_pets = sorted(pets, reverse=True)
>>> new_pets
['Turtle', 'Fish', 'Dingo', 'Cat']
>>> pets.sort(reverse=True)
>>> pets
['Turtle', 'Fish',