map(), filter(), and reduce() in Python with Examples
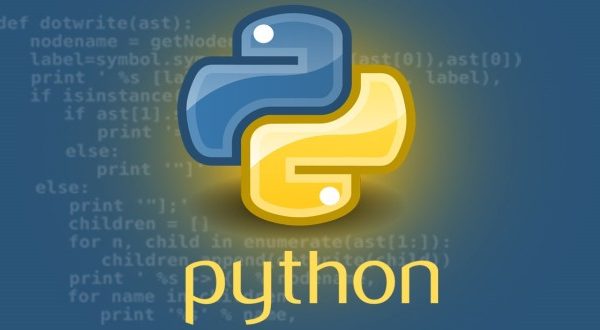
Introduction
The map()
, filter()
and reduce()
functions bring a bit of functional programming to Python. All three of these are convenience functions that can be replaced with List Comprehensions or loops, but provide a more elegant and short-hand approach to some problems.
Before continuing, we’ll go over a few things you should be familiar with before reading about the aforementioned methods:
What is an anonymous function/method or lambda?
An anonymous method is a method without a name, i.e. not bound to an identifier like when we define a method using def method:
.
Note: Though most people use the terms “anonymous function” and “lambda function” interchangeably – they’re not the same. This mistake happens because in most programming languages lambdas are anonymous and all anonymous functions are lambdas. This is also the case in Python. Thus, we won’t go into this distinction further in this article.
What is the syntax of a lambda function (or lambda operator)?
lambda arguments: expression
Think of lambdas as one-line methods without a name. They work practically the same as any other method in Python, for example:
def add(x,y):
return x + y